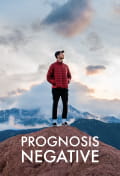
Prognosis Negative
- Star rating
- 2.66
- Rating
- PG-13
- Year
- 2021
- Genre
- Comedy
- Runtime
- 1h 46m
- Cast
- Simon Pegg, Zach Galifianakis
Design
first, Start a new API journey with highly Descriptive
, Modularized
and Visualized
experience. Integrate Distribution
, Monitoring
, and Management
of Your API Assets.
syntax = "proto3";
service OrderService {
option (hope.swagger.svc) =
{ path: "/order"; description: "Order Service"; }
rpc Place (PlaceOrderRequest)
returns (OrderPlacedResponse) {
option (hope.swagger.operation) = {
get: "/place";
description: "place a new order";
};
}
rpc Query (QueryOrderRequest)
returns (OrderView) {
option (hope.swagger.operation) = {
get: "/query";
pageable: true;
description: "query all live order";
};
}
}
Full language support in IDEA.
Get intelligent autocomplete suggestions, linting, syntax highlighting;
All within your favorite editor with plugin: The ApiHug - API design Copilot
.
Easy integration with your toolchain.
Extend Gradle to handle projects of any scale, from single modules to enterprise-level multi-module projects.
Experience faster excellence in building, automating, and delivering with ease and efficiency.
1syntax = "proto3";23service OrderService {45 rpc Place (PlaceOrderRequest)6 returns (OrderPlacedResponse) {7 option (hope.swagger.operation) = {8 get: "/place";9 10 description: "place a new order";11 };12 }13}14
gradlew clean build -x test -w -x stubTest
Efficient Development.
Module Sharing, Version Control, API Repository.
Standard API Design Meta Language, Specification-driven, Single Source of Truth, Unified IDE for More Efficient Collaboration.
syntax = "proto3";
import "";
message Movie {
string name = 1 [(hope.swagger.field) = {
description: "name of the movie";
example: "The Lord of the Rings"
empty: FALSE;
mock: { nature: MOVIE }
max_length: { value: 64 }
}];
MovieLevel level = 2 [(hope.swagger.field) = {
description: "level of the movie";
example: "PG_13"
empty: FALSE;
}];
uint32 year = 3 [(hope.swagger.field) = {
description: "publish year";
example: "2022"
}];
}
Intuitive Design + Efficient Code Generation = Elegant Database Design;
Comprehensive Coverage from API to Data Access.
syntax = "proto3";
message Movie {
string name = 1 [(hope.persistence.column) = {
name: "NAME", description: "Name of the movie",
type: VARCHAR, length: { value: 64}
}];
string description = 2 [(hope.persistence.column) = {
name: "DESCRIPTION", description: "description of the movie",
type: VARCHAR, length: { value: 255 }
}];
MovieLevel level = 3 [(hope.persistence.column) = {
name: "LEVEL", enum_type: STRING,
type: VARCHAR, length: { value: 16 }
}];
option (hope.persistence.table) = {
name: "MOVIE", description: "Movie",
wires: [IDENTIFIABLE, AUDITABLE]
};
}
<createTable remarks="Movie" tableName="MOVIE"> <column name="ID" type="BIGINT" autoIncrement="true"> <constraints nullable="false" primaryKey="true" unique="true"></constraints> </column> <column name="NAME" type="VARCHAR(64)" remarks="name of the movie"> <constraints nullable="false"></constraints> </column> <column name="DESCRIPTION" type="VARCHAR(255)" remarks="description of the movie"> <constraints nullable="false"></constraints> </column> <column name="CREATED" type="TIMESTAMP" remarks="Record deleted at"></column></createTable>
Move even faster with ApiHug.
Rapidly develop enterprise-grade components with pro developer tools, intuitive designers, and code generation.
Our modern, lightweight Java framework maximizes developer performance in a connected, robust enterprise platform.